In computer programming, especially in C programming, loops play an essential role in controlling the flow of execution. The ‘Do While Loop in C’ is one of the fundamental loop structures, offering a unique way to handle repetitive tasks. Unlike other loops, a do while loop guarantees that the loop body will be executed at least once, as the condition is evaluated at the end of the loop. This makes it particularly useful in scenarios where the loop must run at least once regardless of the condition. Understanding its syntax and application is key for anyone delving into C programming.
Understanding the Syntax of Do While Loop
The syntax of the do while loop in C is straightforward yet distinct. It begins with the do
keyword, followed by the loop body enclosed in curly braces. After the loop body, the while
keyword appears, with the loop continuation condition enclosed in parentheses. The loop terminates with a semicolon. This loop structure is a part of the broader loop syntax spectrum in C programming and is known for its simplicity and effectiveness in various programming scenarios, from algorithms in computer science to applications in big data and computer networks.
Basic Syntax:
- Start with the
do
keyword. - Enclose the loop body within curly braces
{}
. - Use the
while
keyword followed by the condition. - End the loop with a semicolon.
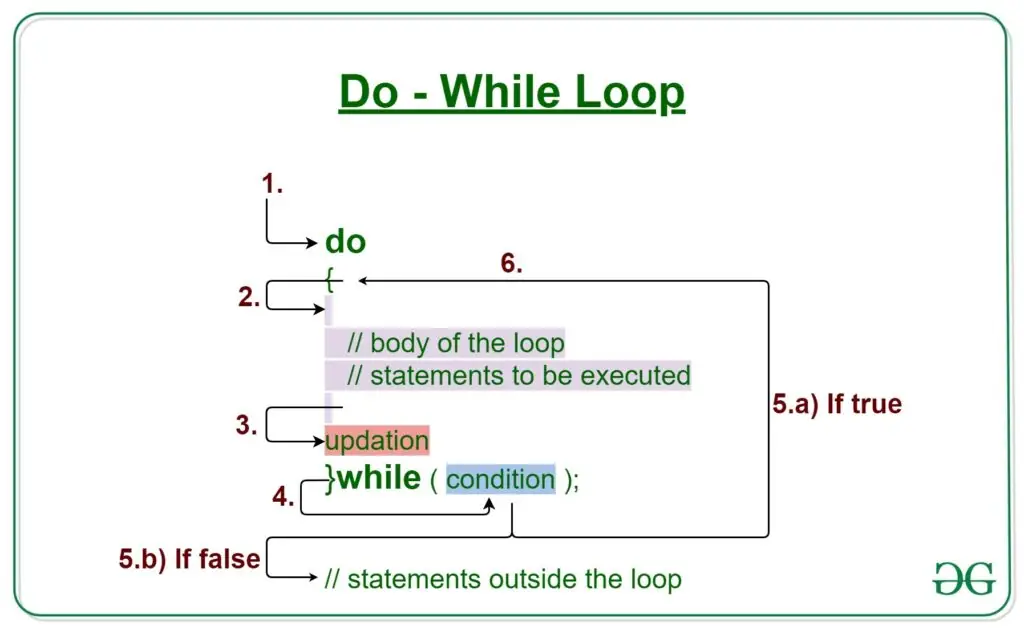
Role of Loop Control Variable
In a do while loop, the loop control variable is pivotal. It is generally initialized before the loop starts, and its value is used and updated within the loop. The initialization sets the starting point of the loop, while the update operation, typically at the end of the loop body, alters the loop control variable’s value. This update is crucial for eventually meeting the loop termination condition and avoiding an infinite loop. The loop control variable’s role is to maintain the loop’s progress and ensure that it executes as intended.
Implementing a Do While Loop in C
To implement a do-while loop in C, first initialize the loop control variable. Then, use the do-while
statement followed by a set of braces containing the loop body. After the loop body, write the while
keyword with the loop continuation condition inside the parentheses. This structure allows the loop to execute the code block at least once and then repeatedly as long as the specified condition is true. This makes the do while loop particularly useful in scenarios where the initial execution of the loop body is required regardless of the condition.
Example:
int count = 0;
do {
// Loop body: code to execute
count++;
} while (count < 10);
Differences Between Do While and Other Loops in C
While the do while loop is an integral part of loop control structures in C, it is important to understand how it differs from other loops like the ‘while’ loop. The primary difference lies in when the condition is checked: in a do while loop, the condition is checked after executing the loop body, ensuring at least one execution. In contrast, the ‘while’ loop checks the condition before the loop body, which means the loop body might not execute at all if the condition is initially false. This distinction is crucial for selecting the appropriate loop structure based on the specific requirements of the program.
Avoiding Infinite Loops
One potential pitfall with do while loops is the inadvertent creation of an infinite loop. This occurs when the loop’s condition is always true, resulting in the loop executing indefinitely. To prevent this, ensure that the loop control variable is properly initialized and updated within the loop body. Also, the condition must be designed in such a way that it will eventually become false. Understanding these factors is key in harnessing the power of the do while loop without falling into infinite execution cycles.
Conclusion
The do while loop in C programming is a powerful tool for handling repetitive tasks, with its unique feature of ensuring at least one execution of the loop body. Its syntax is simple yet effective, making it a valuable component in the toolkit of computer programming, especially in C. Understanding its proper usage, differences from other loops, and ways to avoid common pitfalls like infinite loops, is essential for anyone looking to master C programming and its applications in fields like computer organization and architecture, big data, and more. With its straightforward structure and guaranteed execution, the do while loop is an indispensable element in efficient C programming.
FAQ
Related
Follow us on Reddit for more insights and updates.
Comments (0)
Welcome to A*Help comments!
We’re all about debate and discussion at A*Help.
We value the diverse opinions of users, so you may find points of view that you don’t agree with. And that’s cool. However, there are certain things we’re not OK with: attempts to manipulate our data in any way, for example, or the posting of discriminative, offensive, hateful, or disparaging material.