When it comes to programming in C++, there are various mathematical operations that you might need to perform. One such operation is squaring a number.
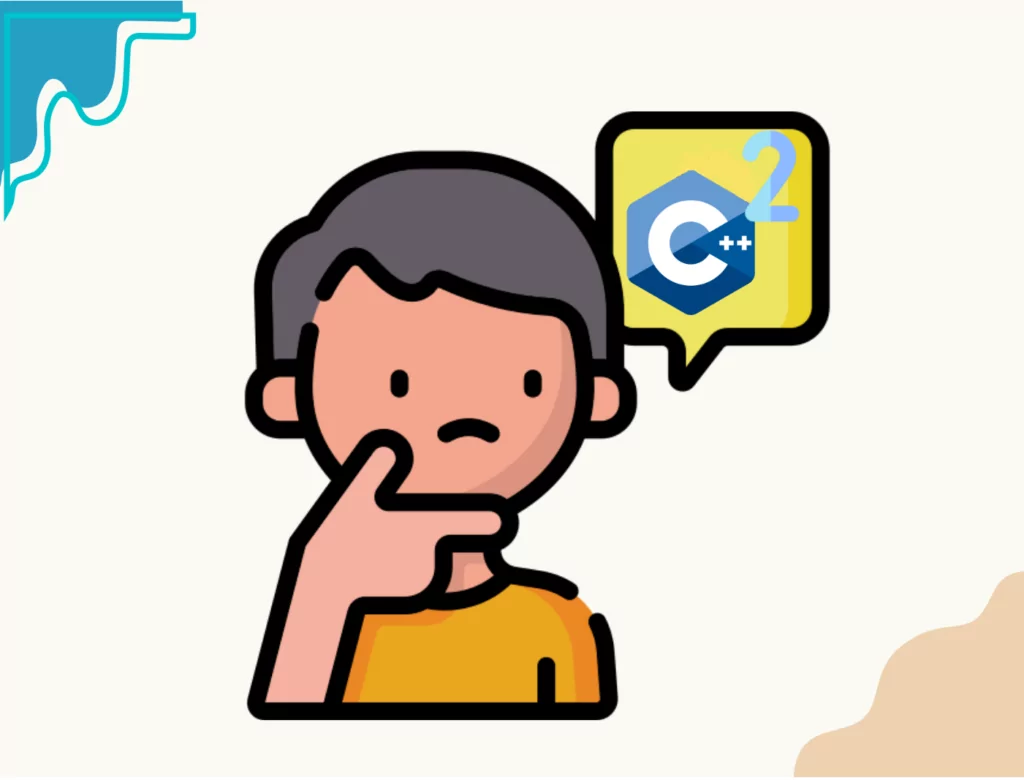
Squaring a number simply means multiplying it by itself. In this guide, we will explore different methods to square a number in C++, along with useful code examples. So, let’s dive in and discover how to square a number in C++ effectively.
Method 1: Using the Power Function
The power function in C++ allows us to raise a number to a specific power. To square a number, we can use the power function and set the power value to 2. Let’s take a look at the code snippet below:
#include <iostream>
#include <cmath>
using namespace std;
int main(){
int number = 5;
float square = pow(number, 2);
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
In the above code, we include the necessary header files, i.e., <iostream>
and <cmath>
, which provide us with the required functionalities. We declare a variable number
and initialize it with the desired value. Then, using the pow()
function, we calculate the square of the number and store it in the square
variable. Finally, we display the result using the cout
statement.
By using this method, we can easily obtain the square of a number in C++.
Method 2: Utilizing a For Loop
Another approach to calculate the square of a number is by using a for loop. This method allows us to perform addition repeatedly to obtain the square. Let’s consider the following code example:
#include <iostream>
using namespace std;
float calculateSquare(float number){
float result = 0.0;
for(int i = 0; i < number; i++){
result += number;
}
return result;
}
int main(){
float number = 5.0;
float square = calculateSquare(number);
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
In the above code, we define a function calculateSquare()
that takes a floating-point number as input. Inside the function, we initialize a variable result
to store the final square value. Using a for loop, we repeatedly add the number to itself. The loop iterates number
times, incrementing i
each time. Finally, we return the calculated square value.
By employing this method, we can obtain the square of any given number without directly using multiplication or division.
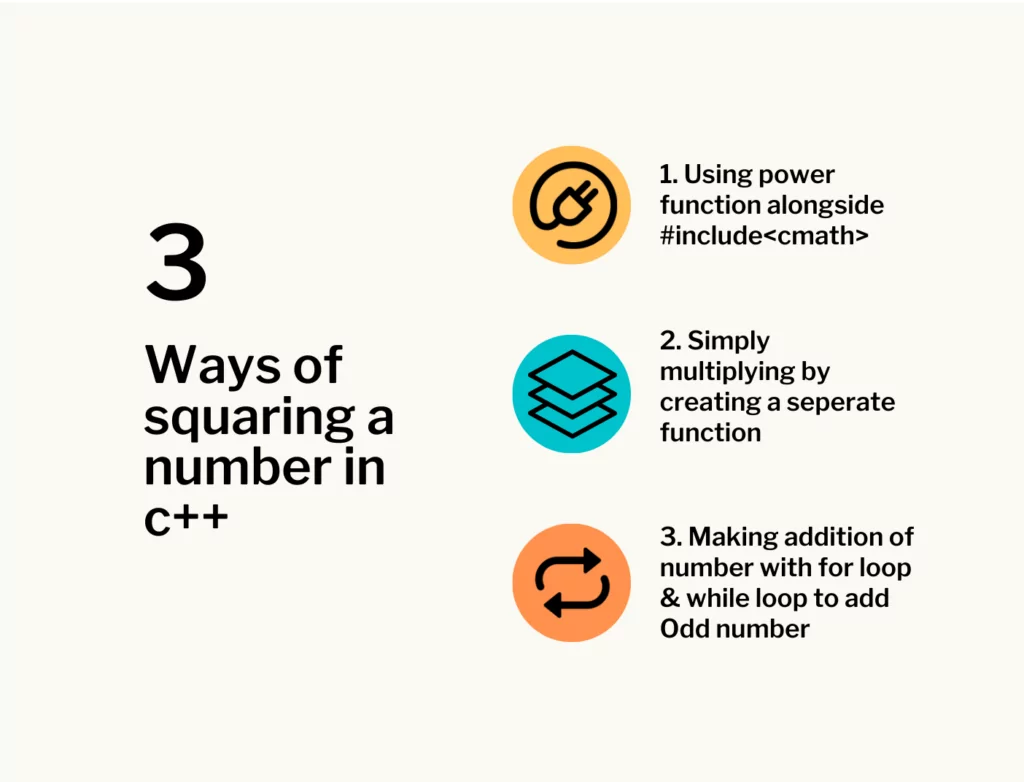
Method 3: Implementing a While Loop
The third method to square a number involves utilizing a while loop. This approach is slightly different from the previous methods and requires the inclusion of an odd number to ensure the square is calculated correctly. Let’s take a look at the following code example:
#include <iostream>
using namespace std;
float calculateSquare(float number){
float oddNum = 1.0;
float squareNum = 0.0;
number = abs(number);
while(number--){
squareNum += oddNum;
oddNum += 2;
}
return squareNum;
}
int main(){
float number = 5.0;
float square = calculateSquare(number);
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
In the above code, we define a function calculateSquare()
that takes a floating-point number as input. Inside the function, we initialize two variables, oddNum
and squareNum
, to keep track of the calculations. We also apply the abs()
function to ensure we’re working with the positive value of the input number.
Using a while loop, we iterate until the number
variable reaches zero. In each iteration, we add the oddNum
value to the squareNum
variable and increment oddNum
by 2. This ensures that we’re adding odd numbers in the process. Finally, we return the square value.
By using this method, we can obtain the square of any number using a while loop.
Conclusion
In this article, we explored different methods to square a number in C++. We started by using the power function, which allows us to raise a number to a specific power. Then, we discussed how to utilize a for loop and a while loop to calculate the square of a number by performing repeated addition.
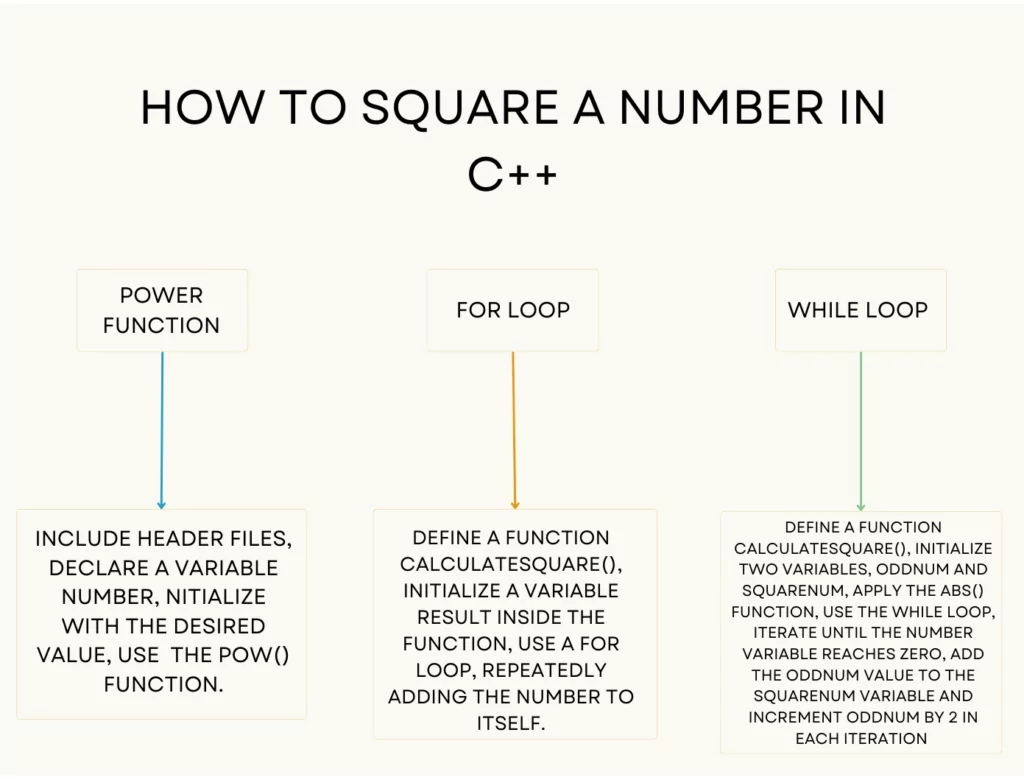
By mastering these techniques, you can easily incorporate square operations into your C++ programs. Whether you need to perform complex calculations or solve mathematical problems, the ability to square numbers is an essential skill.
FAQ
Can I use the pow() function to square a number in C++?
Yes, you can use the pow()
function to square a number in C++. The pow()
function is part of the <cmath>
library, and it allows you to raise a number to a specific power. To square a number, set the power value to 2. Here’s an example of how to use pow()
to square a number:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int number = 5;
float square = pow(number, 2);
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
Is there a for loop method to find the square of a number in C++?
Yes, there is a for loop method to find the square of a number in C++. Instead of using the pow()
function, you can utilize a for loop to perform repeated addition to obtain the square. Here’s an example of the for loop method:
#include <iostream>
using namespace std;
float calculateSquare(float number){
float result = 0.0;
for(int i = 0; i < number; i++){
result += number;
}
return result;
}
int main(){
float number = 5.0;
float square = calculateSquare(number);
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
What is the while loop approach for squaring a number in C++?
The while loop approach for squaring a number in C++ involves using an odd number to ensure the square is calculated correctly. The loop iterates until the number reaches zero, adding odd numbers in each iteration. Here’s an example of the while loop method:
#include <iostream>
using namespace std;
float calculateSquare(float number){
float oddNum = 1.0;
float squareNum = 0.0;
number = abs(number);
while(number--){
squareNum += oddNum;
oddNum += 2;
}
return squareNum;
}
int main(){
float number = 5.0;
float square = calculateSquare(number);
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
Are there any other mathematical functions to square a number in C++?
Yes, there are other mathematical functions that can be used to square a number in C++. Apart from pow()
, you can also use the *
operator for direct multiplication to find the square. Here’s an example:
#include <iostream>
using namespace std;
int main() {
int number = 5;
int square = number * number;
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
How do I handle negative numbers when squaring in C++?
When squaring negative numbers in C++, you need to ensure that the result is positive, as squaring any number produces a non-negative result. To handle negative numbers, you can use the abs()
function from the <cmath>
library to convert negative values to their positive equivalents before performing the square operation. Here’s an example:
#include <iostream>
#include <cmath>
using namespace std;
int main() {
int number = -5;
int square = pow(abs(number), 2);
cout << "The square of " << number << " is: " << square << endl;
return 0;
}
What data types are suitable for storing the square of a number in C++?
The data type suitable for storing the square of a number in C++ depends on the range of values you expect for the square. If you are squaring integers, you can use int
or long long int
data types depending on the range of the numbers. For decimal or floating-point numbers, you can use float
or double
data types. It’s essential to choose a data type that can accommodate the potential magnitude of the squared value without losing precision.
Can I use the square of a number for any specific applications in C++?
Yes, the square of a number finds applications in various fields. For instance, in geometry, the square of the side length is used to find the area of a square. In physics, it plays a significant role in calculating kinetic energy or distance. In computer graphics and gaming, squaring numbers is useful for position calculations. Additionally, squared values are employed in statistical analysis, optimization algorithms, and cryptography, among other domains.
In conclusion, understanding different methods to square numbers in C++ and applying them based on the specific use case can greatly enhance your programming capabilities and enable you to tackle a wide range of mathematical problems.
Follow us on Reddit for more insights and updates.
Comments (0)
Welcome to A*Help comments!
We’re all about debate and discussion at A*Help.
We value the diverse opinions of users, so you may find points of view that you don’t agree with. And that’s cool. However, there are certain things we’re not OK with: attempts to manipulate our data in any way, for example, or the posting of discriminative, offensive, hateful, or disparaging material.