In the world of Java programming, parsing plays a crucial role in extracting essential information from textual data. Whether you are dealing with user input, file processing, or data manipulation, parsing is a fundamental concept to master. In this guide, we will explore the concept of parsing in Java and delve into the various parse() methods available in Java classes. So let’s dive in!
Understanding Parsing in Java
Parsing, in its most general sense, refers to the extraction of necessary information from a given piece of data, usually in the form of text. In Java, many classes provide parse() methods that allow developers to extract and convert data into appropriate objects. These parse() methods receive a string as input, extract the required information, and convert it into an object of the calling class.
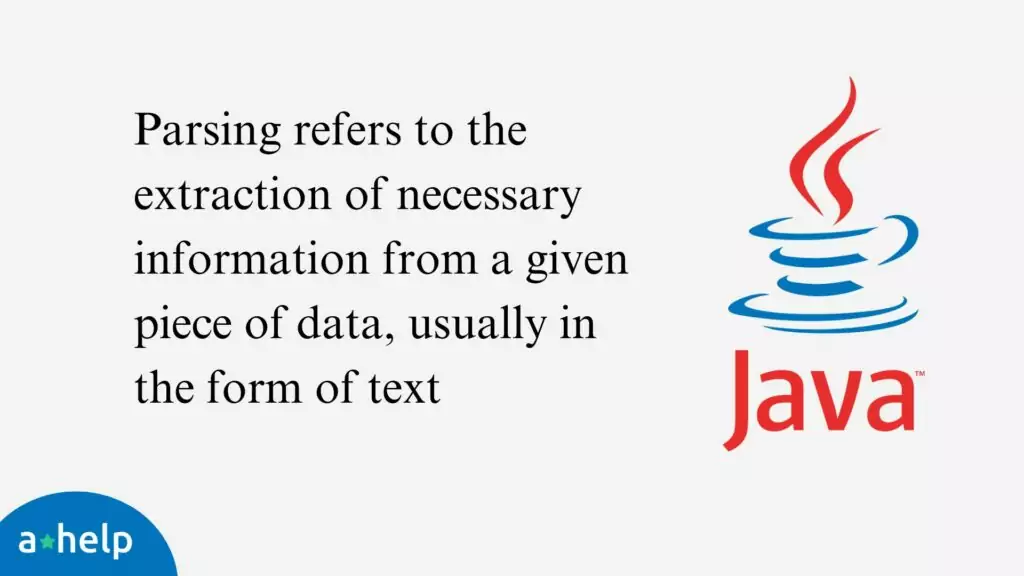
The parseInt() Method
One of the most popular parse() methods in Java is parseInt(). This method is used to extract a primitive data type from a string, specifically converting a string to a number. The parseInt() method can take one or two arguments.
The syntax of parseInt() is as follows:
static int parseInt(String s)
static int parseInt(String s, int radix)
In the first syntax, the string “s” represents a signed decimal value. The second syntax allows specifying the radix, which represents the base of a numerical system. It’s important to note that the radix parameter is optional, and if not provided, the default base value is assumed to be within the range of 2 and 36.
Here’s an example that demonstrates how to use parseInt() in Java:
public class ParseIntExample {
public static void main(String[] args) {
int x = Integer.parseInt("12");
double c = Double.parseDouble("12");
int b = Integer.parseInt("100", 2);
System.out.println(Integer.parseInt("12"));
System.out.println(Double.parseDouble("12"));
System.out.println(Integer.parseInt("100", 2));
System.out.println(Integer.parseInt("101", 8));
}
}
The output of the above code will be:
12
12.0
4
65
The Period parse() Method
The Period class in Java is used to model a quantity of time in terms of years, months, and days. It provides a parse() method to obtain a period from a text representation.
The syntax of the period parse() method is as follows:
public static Period parse(CharSequence text)
The parse() method accepts a CharSequence, which can be implemented by Strings. Therefore, you can use strings as input for the parse() method. However, it’s important to ensure that the string is in the proper format to return an object of the Period class.
The format for the period representation is “PnYnMnD”, where “Y” represents years, “M” represents months, and “D” represents days. The corresponding numbers represent the values for each period.
Here’s an example that demonstrates using the period parse() method in a real-world context:
import java.time.Period;
public class ParseDemo1 {
public static void main(String[] args) {
String age = "P17Y9M5D";
Period p = Period.parse(age);
System.out.println("The age is:");
System.out.println(p.getYears() + " Years\n"
+ p.getMonths() + " Months\n"
+ p.getDays() + " Days\n");
}
}
The output of the above code will be:
The age is:
17 Years
9 Months
5 Days
The SimpleDateFormat parse() Method
The SimpleDateFormat class in Java is used for formatting and parsing dates in a locale-sensitive manner. It provides the parse() method, which breaks down a string into date tokens and returns a Date value in the corresponding format. The parsing starts at the index specified by the developer.
The syntax of the SimpleDateFormat parse() method is as follows:
public Date parse(String the_text, ParsePosition position)
This method takes two parameters. The first parameter is the string to be parsed, and the second parameter is the starting index for parsing. If the position parameter is not provided, the parsing starts from the zero index.
Here’s an example that demonstrates the implementation of the SimpleDateFormat parse() method:
import java.text.ParseException;
import java.text.ParsePosition;
import java.text.SimpleDateFormat;
import java.util.Date;
public class ParseDemo2 {
public static void main(String[] args) throws ParseException {
SimpleDateFormat simpleDateFormat1 = new SimpleDateFormat("MM/dd/yyyy");
SimpleDateFormat simpleDateFormat2 = new SimpleDateFormat("dd/MM/yyyy");
Date date1 = simpleDateFormat1.parse("01/14/2020");
System.out.println(date1);
Date date2 = simpleDateFormat2.parse("14/10/2020");
System.out.println(date2);
ParsePosition p1 = new ParsePosition(18);
ParsePosition p2 = new ParsePosition(19);
ParsePosition p3 = new ParsePosition(5);
String myString = "here is the date: 14/010/2020";
Date date3 = simpleDateFormat2.parse(myString, p1);
Date date4 = simpleDateFormat2.parse(myString, p2);
Date date5 = simpleDateFormat2.parse(myString, p3);
System.out.println(date3);
System.out.println(date4);
The output of the above code will be:
Tue Jan 14 00:00:00 GMT 2020
Wed Oct 14 00:00:00 GMT 2020
null
Wed Oct 14 00:00:00 GMT 2020
null
The LocalDate parse() Method
The LocalDate class is part of the Java 8 Date and Time API (java.time) and represents a date without a time component. It provides a parse() method to convert a string into a LocalDate object.
The LocalDate parse() method has two variants, both of which help convert a string into a LocalDate object.
The first variant of the parse() method takes a string and a formatter as parameters:
The LocalDate parse() Method
The LocalDate class is part of the Java 8 Date and Time API (java.time) and represents a date without a time component. It provides a parse() method to convert a string into a LocalDate object.
The LocalDate parse() method has two variants, both of which help convert a string into a LocalDate object.
The first variant of the parse() method takes a string and a formatter as parameters:
public static LocalDate parse(CharSequence text, DateTimeFormatter formatter)
This method requires specifying a formatter to define the pattern of the input string. After parsing, it returns a LocalDate object.
Here’s an example that demonstrates the usage of the LocalDate parse() method with a formatted:
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class ParseDemo3 {
public static void main(String[] args) {
String date = "14/10/2020";
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("dd/MM/yyyy");
LocalDate localDate = LocalDate.parse(date, formatter);
System.out.println("Parsed local date: " + localDate);
System.out.println("Formatted local date: " + formatter.format(localDate));
}
}
The output of the above code will be:
Parsed local date: 2020-10-14
Formatted local date: 14/10/2020
The second variant of the LocalDate parse() method takes a CharSequence (such as a string) as the only parameter:
public static LocalDate parse(CharSequence text)
This method does not require specifying a formatter explicitly. Instead, it uses the DateTimeFormatter.ISO_LOCAL_DATE as the default formatter. It automatically parses the input string based on the ISO 8601 format.
Here’s an example of the value you’ll get after parsing using the default formatted:
2020-04-05
The LocalDate class is commonly used when dealing with date-related operations and calculations. It provides various methods to manipulate and work with dates effectively.
The ZonedDateTime parse() Method
The ZonedDateTime class, also part of the Java 8 Date and Time API, represents a date and time along with the corresponding time zone information. It provides a parse() method to convert a string into a ZonedDateTime object.
The syntax of the ZonedDateTime parse() method is as follows:
public static ZonedDateTime parse(CharSequence text)
This method takes a string as input and returns one or more objects in the ZonedDateTime format. If there is an error during parsing or if the parsing is not possible, the method throws a DateTimeParseException.
Additionally, there is a parse() method with two parameters:
public static ZonedDateTime parse(CharSequence text, DateTimeFormatter formatter)
This method allows obtaining a ZonedDateTime instance from a text value using a specific formatter. If the second parameter is not provided, the DateTimeFormatter.ISO_LOCAL_TIME formatter is used by default.
Let’s take a look at both variants of the ZonedDateTime parse() method:
public static ZonedDateTime parse(CharSequence text)
public static ZonedDateTime parse(CharSequence text, DateTimeFormatter formatter)
The ZonedDateTime class is particularly useful when dealing with time zone-related calculations and representations. It provides extensive functionality for handling date and time values in various time zones.
The LocalTime parse() Method
The LocalTime class represents a time without a date component in the Java 8 Date and Time API. It provides the parse() method to convert a string into a LocalTime object.
The LocalTime parse() method has two variants, each serving a different purpose.
The first variant takes a CharSequence (such as a string) as the only parameter:
public static LocalTime parse(CharSequence text)
This method allows parsing a string without specifying a formatter explicitly. It uses the DateTimeFormatter.ISO_LOCAL_TIME formatter as the default formatter. Therefore, the input string should be in the format “HH:mm:ss” to produce the desired LocalTime object.
The second variant of the parse() method takes a CharSequence and a DateTimeFormatter as parameters:
public static LocalTime parse(CharSequence text, DateTimeFormatter formatter)
This variant enables parsing a string using a specific formatter, allowing for more flexibility in defining the format of the input string.
Here’s an example that demonstrates the usage of both variants of the LocalTime parse() method:
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
public class ParseDemo4 {
public static void main(String[] args) {
String time1 = "10:30:45";
String time2 = "17:45";
LocalTime localTime1 = LocalTime.parse(time1);
LocalTime localTime2 = LocalTime.parse(time2, DateTimeFormatter.ofPattern("HH:mm"));
System.out.println("Parsed local time 1: " + localTime1);
System.out.println("Parsed local time 2: " + localTime2);
}
}
The output of the above code will be:
Parsed local time 1: 10:30:45
Parsed local time 2: 17:45
Both variants of the LocalTime parse() method return a LocalTime object in the format “hh:mm:ss”. However, be aware of DateTimeParseExceptions, which indicate that the format of the input string does not match the expected format for LocalTime objects.
The MessageFormat parse() Method
The MessageFormat class in Java is used for creating and formatting messages that contain dynamically replaced values. It provides the parse() method, which retrieves a string value when given the starting index.
The general syntax of the MessageFormat parse() method is as follows:
public Object[] parse(String source, ParsePosition position)
The parse() method takes two parameters: “source” represents the string to parse, and “position” represents the starting index of parsing.
Here’s an example that demonstrates how to use the MessageFormat parse() method:
import java.text.MessageFormat;
import java.text.ParsePosition;
public class ParseDemo5 {
public static void main(String[] args) {
try {
MessageFormat messageFormat = new MessageFormat("{1, number, #}, {0, number, #.#}, {2, number, #.##}");
ParsePosition pos = new ParsePosition(3);
Object[] result = messageFormat.parse("abc 123.4 def", pos);
System.out.println(result[0]);
System.out.println(result[1]);
System.out.println(result[2]);
} catch (Exception e) {
e.printStackTrace();
}
}
}
The output of the above code will be:
123.4
null
null
The MessageFormat parse() method retrieves values from a given string based on the pattern provided. It inserts the formatted strings into the pattern at the appropriate positions. It’s important to note that if the parsing fails or encounters an error, the method returns null or throws an exception.
The Level parse() Method
In Java’s logging framework, the Level class is used to represent the severity level of a log message. The Level class provides a parse() method that allows developers to parse a string representation of a level and obtain the corresponding Level object.
The syntax of the Level parse() method is as follows:
public static Level parse(String name)
The parse() method takes a string representing the level’s name as its parameter. It can be the name of the level itself, its initializing name, or an integer value. The method returns a Level object corresponding to the provided string.
Here are some commonly used level names in Java’s logging framework:
- SEVERE
- WARNING
- INFO
- CONFIG
- FINE
- FINER
- FINEST
In case the argument contains symbols or values that cannot be parsed, the parse() method throws an IllegalArgumentException.
The Instant parse() Method
The Instant class in Java’s date and time API represents a specific moment in time. It provides the parse() method, which allows developers to parse a string and obtain an Instant object.
The syntax of the Instant parse() method is as follows:
public static Instant parse(CharSequence text)
To parse a string and obtain an Instant, developers need to ensure that the string contains a valid text representation of a date and time. If the provided string is null, the parse() method throws a DateTimeException.
Here’s an example that demonstrates the usage of the Instant parse() method:
import java.time.Instant;
public class ParseDemo6 {
public static void main(String[] args) {
Instant instant = Instant.parse("2020-10-14T10:37:30.00Z");
System.out.println(instant);
}
}
The output of the above code will be:
2020-10-14T10:37:30Z
The Instant parse() method allows developers to convert a string representation of an instant into an Instant object. It’s particularly useful when dealing with high-precision time data.
The NumberFormat parse() Method
The NumberFormat class in Java is used to format and parse numeric values. The parse() method of the NumberFormat class allows developers to convert a string to a number.
Developers often use the parse() method to break down a string into its component numbers. The parsing starts from the beginning of the string. If you call setParseIntegerOnly(true) before calling the parse() method, only the integer part of the number will be converted.
The syntax of the NumberFormat parse() method is as follows:
public Number parse(String str)
The parse() method accepts a string as its parameter and returns a numeric value as the parsing result.
Here’s an example that demonstrates how to use the NumberFormat parse() method:
import java.text.NumberFormat;
public class ParseDemo7 {
public static void main(String[] args) {
try {
NumberFormat numberFormat = NumberFormat.getInstance();
System.out.println(numberFormat.parse("3.141592"));
numberFormat.setParseIntegerOnly(true);
System.out.println(numberFormat.parse("3.141592"));
} catch (Exception e) {
e.printStackTrace();
}
}
}
The output of the above code will be:
3.141592
3
Conclusion
In Java, parsing is the process of extracting necessary information from textual data. Java provides a variety of parse() methods in different classes to convert strings into various data types. Understanding and mastering these parsing methods gives developers flexibility and precision when working with data. By practicing and familiarizing yourself with the syntax and parameters of each method, you can become proficient in parsing data in Java.
In this article, we explored several important parse() methods in Java, including parseInt(), Period parse(), SimpleDateFormat parse(), LocalDate parse(), ZonedDateTime parse(), LocalTime parse(), MessageFormat parse(), Level parse(), Instant parse(), and NumberFormat parse(). Each method has its unique purpose and usage, enabling developers to handle different types of data and scenarios efficiently.
Remember to utilize the appropriate parse() method based on the specific requirements of your Java application. Whether you are parsing dates, numbers, or other types of data, understanding the available parse() methods will empower you to extract and manipulate textual information effectively.
FAQ
How can I parse a string to an integer in Java?
To parse a string to an integer in Java, you can use the parseInt()
method provided by the Integer
class. Here’s an example:
String str = "42";
int number = Integer.parseInt(str);
In this example, the parseInt()
method takes a string as input and returns the corresponding integer value. If the string cannot be parsed into an integer, a NumberFormatException
will be thrown. It’s important to ensure that the string contains a valid representation of an integer before using parseInt()
.
What is the role of exceptions in parsing?
Exceptions play a crucial role in parsing as they help handle errors and exceptional situations during the parsing process. In Java, parsing methods often throw exceptions to indicate when parsing fails or encounters unexpected input.
For example, when parsing a string to a date using SimpleDateFormat.parse()
, a ParseException
is thrown if the string does not match the expected date format. This allows developers to catch and handle the exception gracefully, providing error messages or taking alternative actions.
By using exceptions, developers can handle parsing errors more effectively and ensure that the application handles unexpected input or invalid data gracefully.
Are there any common errors or challenges in parsing Java?
Yes, there are common errors and challenges that developers may encounter when parsing in Java. Some of these include:
- Format mismatches: Parsing requires the input string to match the expected format. If the format is not properly defined or the input string does not adhere to the format, parsing errors can occur.
- Invalid input: When parsing user-provided input, there is always a risk of receiving invalid or unexpected data. Developers need to anticipate and handle such scenarios to avoid application crashes or incorrect results.
- Localization issues: Parsing can be affected by localization settings, such as date and number formats specific to different regions. It’s important to consider localization when parsing input to ensure consistent behavior across different locales.
- Exception handling: As mentioned earlier, parsing methods often throw exceptions to indicate errors. Proper exception handling is essential to handle these exceptions gracefully and provide meaningful feedback to users.
- Performance considerations: In some cases, parsing large amounts of data or using inefficient parsing techniques can impact performance. Developers should strive to optimize parsing operations to improve the overall performance of the application.
What are some best practices for parsing in Java?
When it comes to parsing in Java, following best practices can help ensure efficient and reliable code:
- Validate input: Before parsing, validate the input to ensure it meets the expected format or criteria. This helps prevent errors and unexpected behavior during the parsing process.
- Handle exceptions: Use appropriate exception handling techniques to catch and handle exceptions thrown during parsing. This improves the robustness of the code and provides better error reporting.
- Use specific parsers: Java provides various specialized parsers for different data types, such as
SimpleDateFormat
for dates orNumberFormat
for numbers. Using these specific parsers can simplify the parsing process and handle format-specific details. - Consider localization: Take into account the localization requirements of your application. Use locale-aware parsing methods when dealing with dates, numbers, or other localized data to ensure consistency across different regions.
- Optimize performance: If parsing large amounts of data or performing frequent parsing operations, consider optimizing the code for performance. Use efficient algorithms and techniques to minimize unnecessary parsing and improve overall application speed.
By following these best practices, you can ensure accurate parsing, handle errors effectively, and maintain high-quality code in your Java applications.
Follow us on Reddit for more insights and updates.
Comments (0)
Welcome to A*Help comments!
We’re all about debate and discussion at A*Help.
We value the diverse opinions of users, so you may find points of view that you don’t agree with. And that’s cool. However, there are certain things we’re not OK with: attempts to manipulate our data in any way, for example, or the posting of discriminative, offensive, hateful, or disparaging material.