In modern web development, the ability to encode URLs correctly is crucial for transmitting data over the network and ensuring its accurate interpretation. Java provides powerful tools and libraries to handle URL encoding, allowing developers to adhere to the specification and avoid common pitfalls. In this guide, we will explore the process of URL encoding in Java, covering essential concepts, best practices, and practical examples.
Understanding URL Encoding
URL encoding is the process of translating special characters in a URL into a format that complies with the specification and can be correctly understood and interpreted. It involves replacing reserved characters with their corresponding hexadecimal representation preceded by the ‘%’ symbol. This ensures that URLs are safe for transmission and can be properly parsed by web servers and browsers.
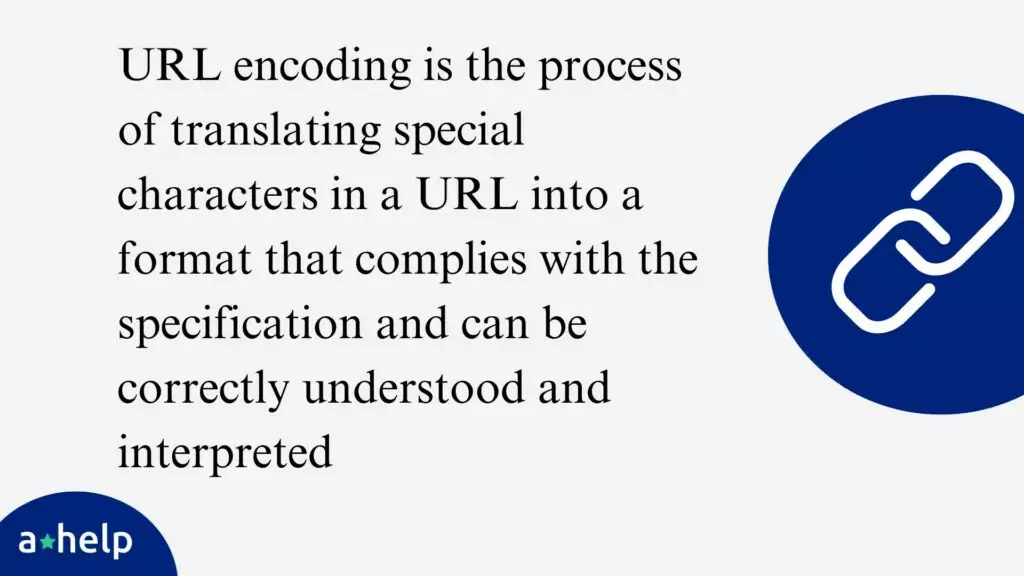
Analyzing the URL
Before diving into the encoding process, it’s essential to analyze the URL and identify the relevant portions that need encoding. A URL consists of various components, such as the scheme, host, path, query parameters, and fragments. By understanding the structure of the URL, we can determine which parts require encoding and which parts should remain unchanged.
Let’s consider an example URL: http://www.baeldung.com?key1=value+1&key2=value%40%21%242&key3=value%253
. This URL includes query parameters that may contain special characters. We can utilize the java.net.URI
class to analyze the URL and extract its different components programmatically.
URI uri = new URI(testUrl);
String scheme = uri.getScheme();
String host = uri.getHost();
String query = uri.getRawQuery();
// Extracted components can be further processed or inspected
By using the getScheme()
, getHost()
, and getRawQuery()
methods, we can retrieve the scheme (http
), host (www.baeldung.com
), and raw query parameters (key1=value+1&key2=value%40%21%242&key3=value%253
), respectively.
Encoding the URL
When encoding a URL, it’s crucial to avoid encoding the entire URL. Typically, we only need to encode the query portion of the URL, leaving the scheme, host, and path untouched. Encoding the entire URL may lead to unexpected behavior and non-compliance with the URL specification.
To encode the query parameters, we can use the URLEncoder.encode(value, encodingScheme)
method provided by the java.net.URLEncoder
class. This method accepts the value to be encoded and the desired character encoding scheme.
private String encodeValue(String value) {
return URLEncoder.encode(value, StandardCharsets.UTF_8.toString());
}
// Encoding query parameters
String encodedURL = requestParams.keySet().stream()
.map(key -> key + "=" + encodeValue(requestParams.get(key)))
.collect(joining("&", "http://www.baeldung.com?", ""));
In the above example, we define a helper method encodeValue()
that uses URLEncoder.encode()
to encode each value in the requestParams
map. The resulting encoded values are then concatenated with their respective keys and joined using the joining()
method.
It’s important to note that the World Wide Web Consortium (W3C) recommends using the UTF-8 encoding scheme for URL encoding to ensure compatibility across different systems and platforms.
Decoding the URL
Decoding a URL is the process of reversing the encoding to retrieve the original values. It’s crucial to decode the URL using the same encoding scheme that was used for encoding to avoid data corruption or misinterpretation.
To decode a URL, we can utilize the URLDecoder.decode(value, encodingScheme)
method provided by the java.net.URLDecoder
class. This method takes the encoded value and the corresponding encoding scheme as parameters.
private String decode(String value) {
return URLDecoder.decode(value, StandardCharsets.UTF_8.toString());
}
// Decoding query parameters
URI uri = new URI(testUrl);
String scheme = uri.getScheme();
String host = uri.getHost();
String query = uri.getRawQuery();
String decodedQuery = Arrays.stream(query.split("&"))
.map(param -> param.split("=")[0] + "=" + decode(param.split("=")[1]))
.collect(Collectors.joining("&"));
In the above code snippet, we define a helper method decode()
that uses URLDecoder.decode()
to decode each parameter value in the query string. The decoded values are then concatenated with their respective keys and joined back using the joining()
method.
It’s worth mentioning that proper URL decoding requires analyzing the URL components before decoding. Attempting to decode the URL without analyzing it first may result in incorrect parsing and decoding of the URL portions.
Encoding Path Segments
While the URLEncoder
class can handle URL encoding for query parameters, it should not be used for encoding path segments. Path segments represent the hierarchical structure of a URL and may contain different reserved characters compared to query parameter values.
To encode path segments correctly, we can utilize the UriUtils
class provided by the Spring Framework. It offers encodePath()
and encodePathSegment()
methods specifically designed for encoding path and path segment components, respectively.
private String encodePath(String path) {
try {
path = UriUtils.encodePath(path, "UTF-8");
} catch (UnsupportedEncodingException e) {
LOGGER.error("Error encoding parameter {}", e.getMessage(), e);
}
return path;
}
// Encoding path segment
String pathSegment = "/Path 1/Path+2";
String encodedPathSegment = encodePath(pathSegment);
String decodedPathSegment = UriUtils.decode(encodedPathSegment, "UTF-8");
// Asserting encoded and decoded path segments
assertEquals("/Path%201/Path+2", encodedPathSegment);
assertEquals("/Path 1/Path+2", decodedPathSegment);
In the above example, we define a helper method encodePath()
that uses UriUtils.encodePath()
to encode the given path using the UTF-8 encoding scheme. The encoded path segment can then be used in constructing the final URL.
It’s important to note that certain characters, such as the plus sign (+), are valid in path segments and should not be encoded. The UriUtils
class handles these scenarios correctly, ensuring the integrity of the path structure.
Conclusion
URL encoding is a fundamental aspect of web development, and Java provides powerful tools to handle this process efficiently, including how to use parse in Java for decoding URLs. By understanding the principles of URL encoding and utilizing the appropriate classes and methods, developers can ensure the safe transmission and accurate interpretation of URLs.
You can find more Coding Guides in our designated category here at A*Help!
FAQ
Related
Follow us on Reddit for more insights and updates.
Comments (0)
Welcome to A*Help comments!
We’re all about debate and discussion at A*Help.
We value the diverse opinions of users, so you may find points of view that you don’t agree with. And that’s cool. However, there are certain things we’re not OK with: attempts to manipulate our data in any way, for example, or the posting of discriminative, offensive, hateful, or disparaging material.