When working with Python scripts, there may be times when you need to exit the script programmatically. Whether it’s to handle specific conditions, terminate execution, or ensure proper cleanup, knowing how to exit a Python script is an essential skill for any Python developer. In this guide, we will explore different methods and best practices to exit scripts effectively and offer Python homework assistance for those students who may be stuck.
Understanding the Exit Function
The exit() function in Python provides a straightforward way to exit or terminate a running script or program. It allows you to stop the execution of the program at any point by calling the function. When the exit() function is invoked, the program will immediately halt and exit. Let’s explore how to use the exit() function effectively.
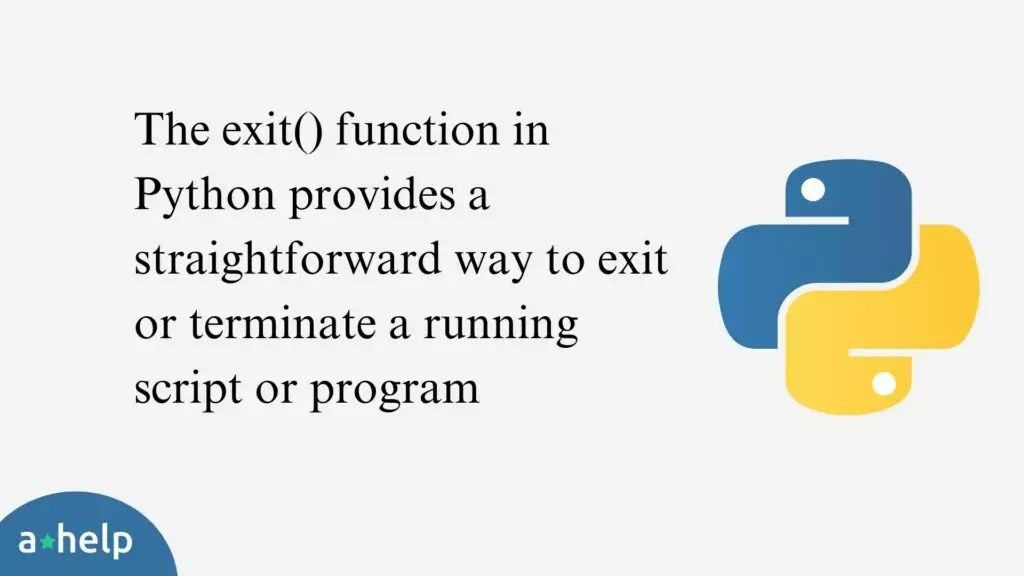
Using the exit() Function in Python
Basic Usage of exit()
To exit a Python script using the exit() function, you simply call the function at the desired exit point. For example, if you want to exit the script after a certain condition is met, you can place the exit() function within an if statement. Here’s an example:
if condition_met:
exit()
Specifying Exit Status
The exit() function also allows you to specify an exit status, which is an integer value indicating the reason for program termination. By convention, a status of 0 represents successful execution, while any non-zero status indicates an error or abnormal termination. You can pass the exit status as an argument to the exit() function. Here’s an example:
if error_occurred:
exit(1) # Terminate the program with status code 1 indicating an error
Handling Exceptions with exit()
In some cases, you may encounter exceptions or errors that require terminating the script. To handle exceptions gracefully and exit the script, you can use a try-except block. Within the except block, you can call the exit() function to terminate the program. Here’s an example:
try:
# Code that may raise an exception
except Exception as e:
print(f"An error occurred: {e}")
exit()
Other Methods to Exit a Python Script
While the exit() function is a common method to exit a Python script, there are alternative approaches you can use. These methods provide similar functionality and can be suitable for different scenarios.
sys.exit()
The sys module in Python provides a function called sys.exit() that can be used to exit a script. This function is similar to the exit() function but allows more flexibility. You need to import the sys module before using sys.exit(). Here’s an example:
import sys
if condition_met:
sys.exit() # Terminate the program
raise SystemExit
Another method to exit a Python script is by raising a SystemExit exception. This exception is designed to terminate the program and can be caught by an except block if needed. Here’s an example:
if condition_met:
raise SystemExit # Terminate the program
Best Practices for Exiting Python Scripts
To ensure clean and effective script termination, it’s important to follow some best practices when using exit() or similar functions. Here are some guidelines to consider:
Importing the sys Module
Before using the exit() function or sys.exit(), remember to import the sys module at the beginning of your script. This allows access to the necessary functions for script termination.
Determining the Exit Condition
Identify the condition or situation that warrants script termination. This can be based on user input, specific events, error conditions, or any other criteria that require the program to stop. Plan your script’s logic accordingly.
Proper Resource Cleanup
If your script utilizes resources such as open files or network connections, it’s good practice to clean up those resources before terminating the script. This ensures that resources are properly handled, even if the program exits unexpectedly. Include cleanup code before calling exit() or sys.exit().
Documenting Exit Conditions
To improve code readability and maintainability, document the specific exit conditions in your script. Use comments to indicate why the program is being terminated at a particular point. This helps other developers understand the purpose and behavior of exit calls.
Conclusion
In this comprehensive guide, we have explored different methods and best practices for exiting a Python script. We have covered the usage of the exit() function, alternative approaches like sys.exit() and raise SystemExit, and discussed important considerations for clean script termination.
By understanding how to exit a Python script effectively, you can handle various scenarios, such as error handling, conditional termination, testing and debugging, and script completion. Remember to import the necessary modules, determine the exit conditions, clean up resources if necessary, and document your code for clarity.
Exiting a Python script when needed ensures proper program flow and maintains code integrity. By following the guidelines outlined in this guide, you can become proficient in exiting Python scripts and handle program termination with confidence.
FAQ
What is the exit function in Python?
The exit() function in Python is a built-in function that is used to exit or terminate the current running script or program. It allows you to stop the execution of the program at any point. By calling the exit() function, the program will immediately halt and exit.
Can I stop the execution of a Python program at any point?
Yes, you can stop the execution of a Python program at any point by using the exit() function. This function provides a convenient way to terminate the program based on specific conditions or events. By calling exit(), you can ensure that the program stops running and exits immediately.
How do I use the exit() function in Python?
To use the exit() function in Python, you simply call the function at the desired exit point in your code. The basic usage of exit() involves invoking it without any arguments, which will terminate the program immediately. However, you can also specify an exit status code as an optional argument to indicate the reason for program termination.
What happens when the exit() function is called in a Python script?
When the exit() function is called in a Python script, several things happen. First, the program execution stops immediately at the point where the exit() function is invoked. The remaining code after the exit() call will not be executed. The script will then exit, returning control to the operating system.
Additionally, you have the option to provide an exit status code as an argument to the exit() function. This exit status code is an integer value that indicates the reason for program termination. By convention, a status of 0 indicates successful execution, while any non-zero status represents an error or abnormal termination.
The exit() function is a powerful tool to control the flow of your Python programs and handle various termination scenarios effectively.
Follow us on Reddit for more insights and updates.
Comments (0)
Welcome to A*Help comments!
We’re all about debate and discussion at A*Help.
We value the diverse opinions of users, so you may find points of view that you don’t agree with. And that’s cool. However, there are certain things we’re not OK with: attempts to manipulate our data in any way, for example, or the posting of discriminative, offensive, hateful, or disparaging material.